Vue Js Check if String is Empty:In Vue.js, you can check if a string is empty using the v-if
directive along with the trim
method. The trim
method removes any whitespace from the beginning and end of the string, leaving only its content. You can then use the length
property to check if the resulting string is empty.
How can you check if a string is empty in Vue js?
The Below code uses Vue.js to check if a string is empty. The v-if
directive is used to conditionally render the first <p>
element if the length of the trimmed string is 0 (i.e., the string contains only whitespace), and the v-else
directive is used to render the second <p>
element if the string is not empty.
The trim()
method is used to remove any leading and trailing whitespace from the string, and the length
property is used to determine the length of the resulting string.
Vue Js Check if String is Empty Example
<div id="app">
<p v-if="myString.trim().length === 0">The string is empty.</p>
<p v-else>The string is not empty.</p>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
myString: ' ', // Set the initial value of the string to an empty string
};
},
})
</script>
Output of Vue Js Check if String is Empty
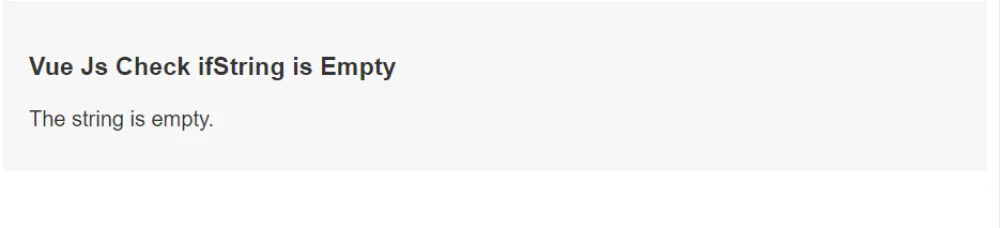